Recently I was looking for a way to display a category list in WordPress with icons in front of each category name, to get a result like this:
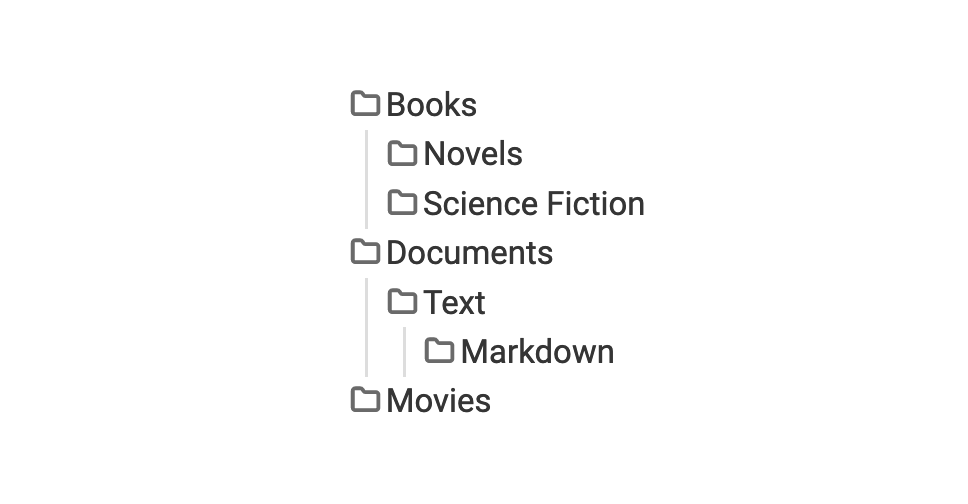
The standard wp_list_categories
function does not offer this possibility, but there is an easy way to get this result using a custom walker. The walker class helps to parse and modify a hierarchical structure generated by WordPress functions like wp_list_categories
. Using a walker we can create the list ourselves and add an icon in front of the link (I’m using file_get_contents
to create an inline svg element, but any html content can be added):
class Icon_Category_Walker extends Walker_Category {
// Override the start_el method to add the SVG icon.
function start_el(&$output, $category, $depth = 0, $args = array(), $id = 0) {
$output .= '<li class="categories-list-item">';
$icon = file_get_contents( get_template_directory_uri() . '/img/icons/folder.svg' ); // add the icon on this line
$output .= '<a href="' . esc_url(get_category_link($category->term_id)) . '">' . $icon . $category->name . '</a>';
}
// Override the end_el method to close the list item.
function end_el(&$output, $page, $depth = 0, $args = array()) {
$output .= '</li>';
}
}
After adding Icon_Category_Walker
to functions.php
, the custom walker can be used when displaying the categories. This is done by adding 'walker' => new Icon_Category_Walker
as an argument of wp_list_categories
:
$args = array(
'echo' => true,
'walker' => new Icon_Category_Walker(),
'hierarchical' => true,
// more...
);
wp_list_categories( $args );
Now (with some styling of the list) the category list should look like in the example above.